If you want to add and manage software licenses in Node JS applications, there are several different options you can choose. You can either create a simple license key generator that works for small-scale use, or implement a software licensing solution for your Node JS app that works in the long run. In this post, we will briefly cover these two alternatives.
License Key Generator – A Shortcut to Software Licensing
The simple way to implement software licensing for your Node JS project is to have a script run partial key verification and create subsets of license keys that you hand out to your customers. However, the structure of these license keys will start to leak over time. The cost of developing and maintaining your own system can also make this option unattractive for long-term and more serious use cases. For more information about license key generators, please read this blog post.
Manage Software Licensing with a Licensing as a Service (LaaS) Provider
Instead of having to code an entire software licensing system yourself, you can work with a Licensing as a Service (LaaS) provider such as Cryptolens to outsource licensing to experts. Then, you get full access to our advanced licensing techniques from day 1, at a fraction of the price of coding and maintaining such a system yourself.
The easiest way to manage software licenses in Node JS applications is by installing our NodeJS SDK, available open source on GitHub. The SDK is available as a NPM package. Then, you can simply sign up to our platform for free, and paste a short code snippet into your Node JS code. For our full documentation page, please visit this page.
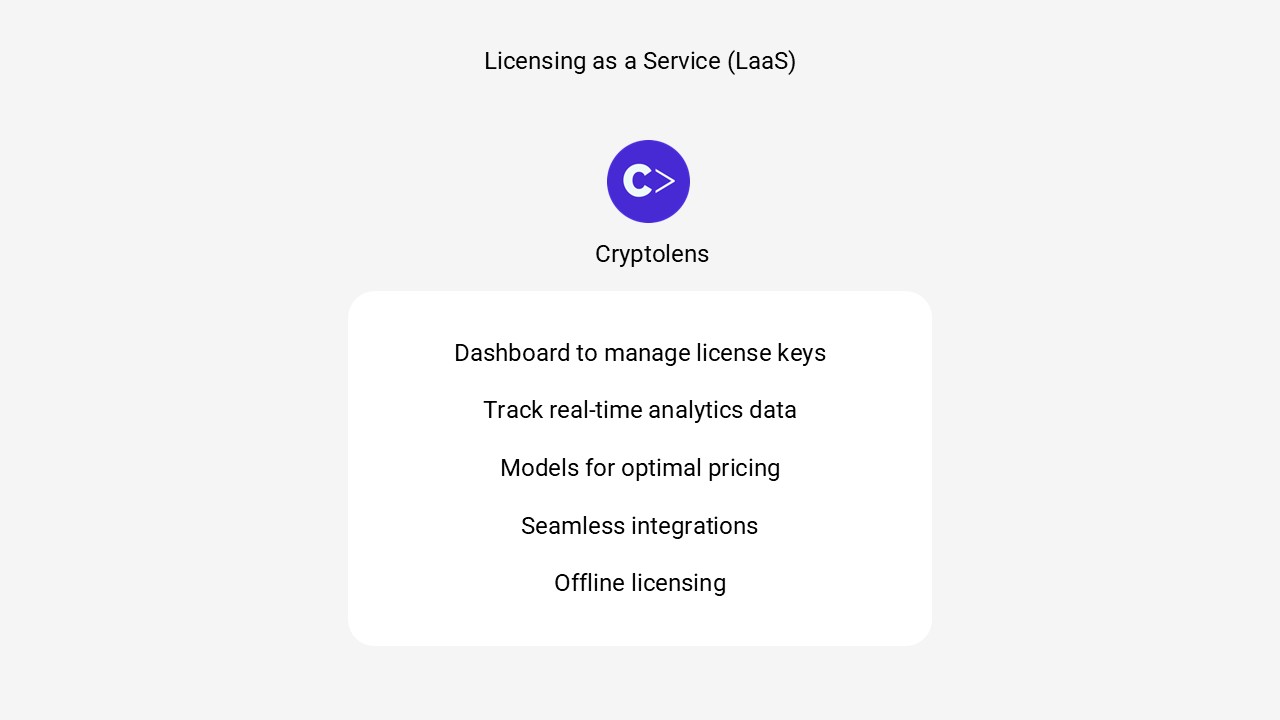
The code snippet below verifies a license key with our API. This example is covered in more detail on this page. If your application needs to work offline, please use this code snippet instead.
const key = require('cryptolens').Key; const Helpers = require('cryptolens').Helpers; var RSAPubKey = "Your RSA Public key, which can be found here: https://app.cryptolens.io/User/Security"; var result = key.Activate(token="Access token with with Activate permission", RSAPubKey, ProductId=3349, Key="GEBNC-WZZJD-VJIHG-GCMVD", MachineCode=Helpers.GetMachineCode()); result.then(function(license) { // success // Please see https://app.cryptolens.io/docs/api/v3/model/LicenseKey for a complete list of parameters. console.log(license.Created); }).catch(function(error) { // in case of an error, an Error object is returned. console.log(error.message); });
For more information on how to obtain the parameters, please read more here.