Golang License Key Manager
If you want to implement a Golang License Key Manager, there are two main choices to make. A simple way to get started with software licensing in a Golang application is by creating a simple license key generator and a subset of license keys to validate. However, many software vendors quickly rule out that option and instead, opt for a more serious and long-term software licensing solution. In this blog, we will briefly cover the benefits of outsourcing software licensing as opposed to coding your own software license key manager for your Golang product.
Golang License Key Generator – A Shortcut to Licensing
So why is a license key generator not a scalable and long-term solution for Golang apps? Well, a simple self-coded license key generator only performs partial license key verification, and the structure you use to create a subset of license keys will start to leak over time. As a result, this solution is quite easy to bypass. Coding a key generator is usually fine for smaller prototypes and for learning purposes. However, for applications in production, a more long-term and serious software license manager is oftentimes needed. You can read more about this option in this blog post.
Cryptolens – Your Golang License Key Manager
So how do you code a software licensing system that performs full license key verification? As you might expect, coding and maintaining a fully scalable software licensing system will take a lot of time. Many software vendors are instead outsourcing software licensing to a Licensing as a Service (LaaS) provider such as Cryptolens.
Then, you get all of our advanced licensing features in an easy-to-use dashboard from day 1. We support all major licensing models and have detailed guides in Golang, along with other languages. Implementing our cloud-based licensing solution is effortless!
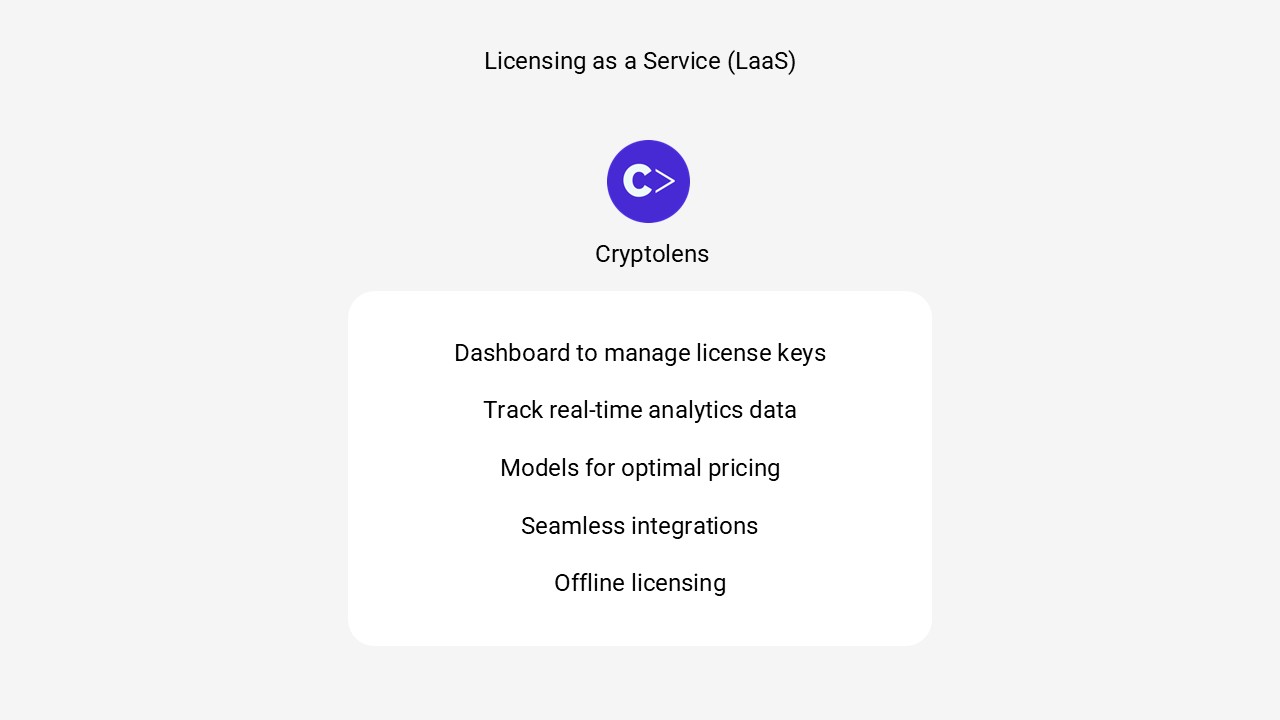
Our Golang library is available on GitHub, and you can sign up to our platform for free. Then, you have to paste a short code snippet into your Golang code. Our full documentation page is available for reference, but to verify a license key, a similar code to the one below can be used:
token := "Access token"
publicKey := "RSA Public key"
licenseKey, err := cryptolens.KeyActivate(token, cryptolens.KeyActivateArguments{
ProductId: 3646,
Key: "MPDWY-PQAOW-FKSCH-SGAAU",
MachineCode: "289jf2afs3",
})
if err != nil || !licenseKey.HasValidSignature(publicKey) {
fmt.Println("License key activation failed!")
return
}
More examples can be found here.